Identify celestial objects
Contents
Identify celestial objects#
Reminder
Start the exercise with the Python template file in the
src
directory corresponding to the exercise.
Your code must be added to the
main
function.If you write generic functions, put them into libraries to reuse them easily in other applications. Look at Library and Module documentation for more details on Python libraries.
You should carefully follow the division in steps proposed for every exercise and provide specific signatures for each step when this is specified.
Git operations:
Commit very frequently with relevant message. Focus on making clear the reasons why you made the change.
Push once a significant result is obtained.
Code quality:
Check frequently the PyCharm annotations about your code, for example the colored signals in the right vertical gutter.
Execute the
check_commit_status.py
script to check the validation tests.Assess the quality of your code with SonarQube.
Documentation:
Add docstrings (
""" ... """
) at begining of ALL functions & classes.Add one-line comments wherever this is useful to understand your code.
Refer to slides for detailed information on technical topics.
Goal#
This exercise will allow to retrieve from an external database, in our case Simbad, the name of the celestial objects (generally stars) matching the clusters identified in previous exercise.
We convert pixel coordinates of the found clusters to absolute celestial coordinates
Then we submit those celestial coordinates to the remote Simbad database, and we parse the answer to obtain the identified celestial object names.
Principle#
A FITS image contains, in the data block header, the information (metadata) needed to convert the image pixel coordinates (in the image coordinate system) into absolute celestial coordinates. This information consists of a transformation matrix plus some parameters related to the data taking conditions. See WCS slides for a description of celestial coordinates.
The Python module npac.coordinates.py
available in your working area provides various
utilities to exploit these metadata, for example to convert image pixel coordinates
into celestial coordinates in the context of a given FITS image.
There are several global databases and catalogs available that can be accessed as Web services.
We are using one of those (named Simbad), physically located in Strasbourg).
This service can be queried upon, using a normalized client-server Web protocol. In particular,
it can return the name of the celestial object, if any, that correspond to a given sky position.
This protocol is implemented in the Python module stars.py
available in the npac
package.
The previous exercise has been able to identify and measure the pixel clusters of an image. Here, we consider the largest cluster detected previously. We expect that this cluster is associated with an identifiable celestial object, ie. that Simbad can identify.
Implementation details#
As for every exercise, you will import
the modules developed for the previous exercises.
The main program should be written in a file ex5_stars.py
. The main classes and functions
that you will need in this exercise are in the npac.coordinates
module.
Celestial Coordinates#
Celestial coordinates are expressed in RaDec where the coordinates are expressed as two numbers:
The right ascension (Ra)
The declination (Dec)
Typically, the FITS data is just a 2D array, so you need know the observing conditions to reproject it on the sky coordinate system.
To get access to the conversion algorithm, you need to build a dedicated object using the function
get_wcs()
from npac.coordinates
module. This function expects a FITS header
as its argument.
my_wcs = npac.coordinates.get_wcs(fits_header)
Note
You can safely ignore the WARNING messages displayed by FITS when the program is executed.
We summarise below the relationships between different coordinates used in the exercises:
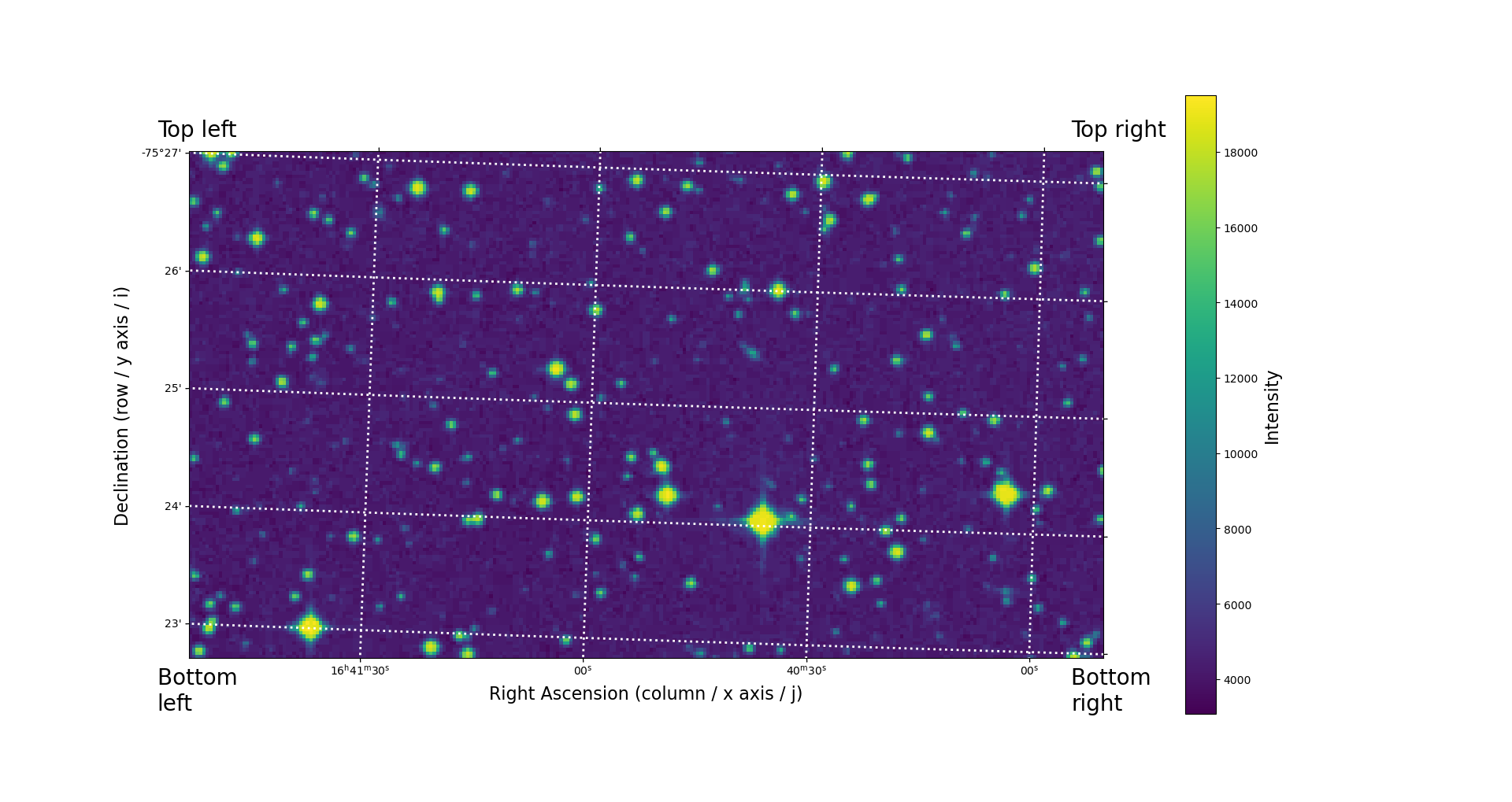
Note that RA and Dec are not parallel to the cartesian axes of your image (as they are coordinates on a sphere).
Querying Simbad#
To query the Simbad database service, you must use get_celestial_objects()
function
from stars
module:
celestial_objects = stars.get_celestial_objects(radec, radius)
The database query arguments are:
radec
: theRaDec
coordinates of the object (in degrees) you want to know the name, expressed as anpac.coordinates.RaDec
object.radius
: this is the acceptance radius which defines the sky region-of-interest around theRaDec
coordinates, expressed in degree. In this exercise, you have to use a value of0.003
degree: it is known to give appropriate results to the queries for the specific image file of yourdata
directory. This value is the default value so that you don’t need to provide it when calling the function.
The query returns a dictionary. If no known celestial object is matching the given sky position with the acceptance radius, the list is empty. Else it will contain one or more entries in which the key is the celestial object identifier and the value is its distance to the observer.
Additional slides and notebooks#
Some slides are needed to complete this document and to get more precise technical information. Sometimes it may even be also needed to explore the complete documentations that are shown in appendices. Here: Fits slides, Pyplot slides, Python notebooks and WCS slides are very useful.
Step 1: coordinate conversion#
The main step to implement the feature described here is:
Using the information given on celestial coordinates, instantiate the conversion object for your image and compute the WCS coordinates of the image corners.
For every cluster detected in the previous exercise, compute the absolute coordinates of its peak.
Signature
This exercise signature starts with the WCS coordinates of the image corners (two floating point values each, for right ascension and declination).
The expected signature format for this exercise is:
signature_fmt_1 = 'RESULT: top_left_corner_right_ascension = {:.6f}' signature_fmt_2 = 'RESULT: top_left_corner_declination = {:.6f}' signature_fmt_3 = 'RESULT: bottom_left_corner_right_ascension = {:.6f}' signature_fmt_4 = 'RESULT: bottom_left_corner_declination = {:.6f}' signature_fmt_5 = 'RESULT: top_right_corner_right_ascension = {:.6f}' signature_fmt_6 = 'RESULT: top_right_corner_declination = {:.6f}' signature_fmt_7 = 'RESULT: bottom_right_corner_right_ascension = {:.6f}' signature_fmt_8 = 'RESULT: bottom_right_corner_declination = {:.6f}'
If you want to check your program with the common image common.fits
, here is
the expected signature:
RESULT: top_left_corner_right_ascension = 250.480671 RESULT: top_left_corner_declination = -75.449980 RESULT: bottom_left_corner_right_ascension = 250.469952 RESULT: bottom_left_corner_declination = -75.378721 RESULT: top_right_corner_right_ascension = 249.967813 RESULT: top_right_corner_declination = -75.454321 RESULT: bottom_right_corner_right_ascension = 249.959537 RESULT: bottom_right_corner_declination = -75.383040
Step 2: highest cluster name#
In this step, we will concentrate on the cluster with the highest integrated luminosity to submit in the Simbad query. Using the information provided above, query the Simbad database and print the query result.
To check that your implementation is correct, after doing it for this cluster (the largest), you may loop over all the clusters identified in your image, retrieve their names if any, and display it. You should verify that the first cluster is indeed associated with one celestial object.
Signature
This exercise signature get the WCS coordinates of the cluster with the highest integrated luminosity, expressed as two floating point values (right ascension and declination), then the name of the closest associated celestial object. The expected signature format for this exercise is:
signature_fmt_9 = 'RESULT: cluster_max_right_ascension = {:.6f}' signature_fmt_10 = 'RESULT: cluster_max_declination = {:.6f}' signature_fmt_11 = 'RESULT: celestial_object = {:s}'
If you want to check your program with the common image common.fits
, here is
the expected signature:
RESULT: cluster_max_right_ascension = 250.152701 RESULT: cluster_max_declination = -75.400437 RESULT: celestial_object = TYC 9443-3715-1